JAVA/WEB - Spring
[AOP] 환경설정 - Maven
개봉박살
2021. 5. 24. 12:32
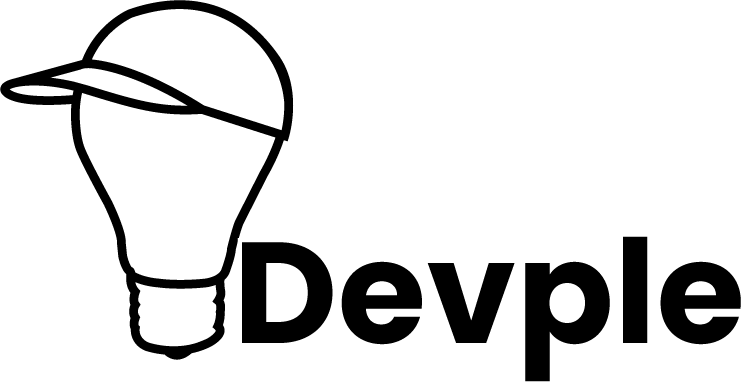
1. depedency
의존성 주입 - pom.xml
<!-- AspectJ --> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjrt</artifactId> <version>1.9.6</version> </dependency> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.9.6</version> </dependency>
2. context설정
config.xml
<?xml version="1.0" encoding="UTF-8"?> <beans:beans xmlns="http://www.springframework.org/schema/mvc" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:beans="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd"> <aop:aspectj-autoproxy/> . . . 생략
3. Aop class
package com.server.study.aop; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.annotation.AfterReturning; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; import org.aspectj.lang.annotation.Pointcut; import org.aspectj.lang.reflect.MethodSignature; import org.springframework.stereotype.Component; import java.lang.reflect.Method; @Aspect @Component public class ParameterAop { //execution 패키지안에 있는 모든 클래스의 모든 메소드에 적용 @Pointcut("execution(* com.server.study.controller..*.*(..))") private void pointCut(){ //com.server.study.controller 안에 있는 모든 클래스의 모든 메서드를 대상으로 삼는다. } // @Pointcut 어노테이션을 적용한 메서드가 실행되기 전에 수행 @Before("pointCut()") public void before(JoinPoint joinPoint){ //실행된 메서드 이름 가져오기 MethodSignature methodSignature = (MethodSignature)joinPoint.getSignature(); String methodName = methodSignature.getMethod().getName(); //아규먼트를 Object 배열로 가져옴 Object[] args = joinPoint.getArgs(); System.out.println(); System.out.println("call method name : "+methodName); System.out.println("================ AOP (before) ================="); for(Object obj : args){ System.out.println(); System.out.println("type: "+obj.getClass().getSimpleName()); System.out.println("value:"+obj.toString()); } System.out.println(); System.out.println("==============================================="); System.out.println(); } // @Pointcut 어노테이션을 적용한 메서드가 예외없이 수행을 마치면 수행 // @After 예외발생에 상관없이 실행 // @AfterReturning 예외없이 수행이 완료되면 실행 // @AfterThrowing 예외 발생 후 실행 @AfterReturning(value="pointCut()", returning="returnObj") public void afterReturn(JoinPoint joinPoint, Object returnObj){ // @AfterReturning 어노테이션의 파라미터 returning 과 // 메소드의 Object 파라미터 이름을 똑같이 맞춰야함 System.out.println(); System.out.println("=-=-=-=-=-=-=-=-=- AOP (after) -=-=-=-=-=-=-=-=-="); System.out.println(); System.out.println("return object"); System.out.println(returnObj); System.out.println(); System.out.println("-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-"); System.out.println(); } }
4. Test Result(Tomcat log)
call method name : select ================ AOP (before) ================= type: Member value:Member(id=1, name=null, email=null, create_date=null) =============================================== Hibernate: select member0_.id as id1_0_0_, member0_.create_date as create_d2_0_0_, member0_.email as email3_0_0_, member0_.name as name4_0_0_ from member member0_ where member0_.id=? =-=-=-=-=-=-=-=-=- AOP (after) -=-=-=-=-=-=-=-=-= return object Member(id=1, name=dean, email=zero2080@naver.com, create_date=2021-05-24T11:17:54.351783) -=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
5. 마치며
AOP는 잘 짜여진 리플렉션의 예제로 보인다. 우리가 자바를 다루면서 한번쯤은 들어봤을 리플렉션은 사실 스프링의 기반이 되며 그것때문에 다양한 기능을 수행하고 사용할수있게 도와준다.
그리고 AOP는 우리가 개발을 할때 기능에 충실한 코드를 작성할 수 있게 도와준다.
쓸대없이 중간에 로그를 출력하는 등의 코드를 비즈니스로직에 섞어 넣지 않아도 된다.
또한 예를 들어 회원가입등의 기능을 수행하기 앞서 AOP @Before 위치에 암호화 모듈을 넣음으로써 좀더 깔끔한 서비스 로직을 짤 수 있다.(물론 Spring Security 필터를 사용하면되지만...)
앞으로 프로젝트 설계 할 때 AOP를 적극 활용하도록하자!!
끝!!